COURSE STRUCTURE
Part 1 - Python
Introduction to Python
- Introductory Remarks about Python
- A Brief History of Python
- How python is differ from other languages
- Python Versions
- Installing Python and Environment Setup
- IDLE
- How to execute Python program
- Writing your first Python program
Variables, Data Types & Operators
- Memory mapping of variables
- Keywords in Python
- Comments in python
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Membership Operators
Data types in Python
- Numbers
- Strings
- Lists
- Tuples
- Dictionary
- Sets
- 3D asset Creation
Numbers and Strings
- Intro. to Python ‘Number’ & ‘string’ data types
- Properties of a string
- String built-in functions
- Programming with strings
- String formatting
Lists and Tuples
- Intro to Python ‘list’ data type
- Properties of a list
- List built-in functions
- Programming with lists
- List comprehensio
Dictionary and Sets
- Intro to Python ‘dictionary’ data type
- Creating a dictionary
- Dictionary built-in functions
- Set and set properties
- Set built-in functions
Decision making & Loops
- Intro of Decision Making
- Control Flow and Syntax
- The if Statement
- The if...else Statement
- The if...elif...else Statement
- Nested if...else Statement
- The while Loop
- break and continue Statement
User defined Functions
- Intro of functions
- Function definition and return
- Function call and reuse
- Function parameters
- Function recipe and docstring
- Scope of variables
- Recursive functions
- Lambda / Anonymous Functions
Modules and Packages
- Module
- Importing module
- Standard Module - sys
- Standard Module - OS
- The dir Function
- Packages
- Exercise
Exception Handling in Python
- Understanding exceptions
- Run Time Errors
- Handling I/O Exceptions
- try, except, else and finally statement
- raising exceptions with: raise, assert
File Handling in Python
- Working with files
- File objects and Modes of file operations
- Reading, writing and use of ‘with’ keyword
- read(), readline(), readlines(), seek(), tell()
- Handling comma separated value files
Regular expression
- Pattern matching
- Meta characters for making patterns
- re flags
- Use of match() , sub() , findall(), search(), split()
Part 2 - Advanced Excel
Intro to MS Excel Environment
- Excel Interface
- Features of MS Excel
- MS Excel functions
- Understanding of data calculations in Excel
- Understanding of Data Tools Panel
- Excel Different Types of Charts Creation
DateTime in Python
- Dashboards
- Designing Sample Dashboard
- Step-by-step Excel dashboard tutorial
- Representation of Dashboard data
- Organizing data in Dashboard
- Tips and Tricks to enhance dashboard designing
Project Work
- Dashboard Analysis Project in Excel
- Stock Management System Project in Excel
Part -3 SQL
Overview of SQL
- SQL
- Database Concepts
- What is Database Package
- Understanding Data Storage
- Understanding of Data Tools Panel
- Relational Database (RDBMS) Concept
Part-1 SQL
- SQL Basic
- SQL Commands
- DDL, TCL, DML & DQL
- DDL: create, alter, drop, rename
- DML: insert, update, delete
- DQL: select
Part-2 SQL
- TCL: rollback, commit
- SQL where
- SQL operators
- SQL like, not like
- SQL between, not between
- SQL order by
Part 4- Data Analytics
PYTHON LIBRARIES
- What is data analysis?
- Why python for data analysis?
- Python Libraries Installation and setup
- Ipython
- Jupyter Notebook
- Relational Database (RDBMS) Concept
NUMPY ARRAYS
- Numpy Arrays
- Numpy Data types
- Numpy Array Indexing
- Numpy Mathematical Operations
- Indexing and slicing
- Manipulating array shapes
WORKING WITH PANDAS
- Data structure of pandas
- Pandas Series
- Pandas dataframes
- Data aggregation with Pandas
- DataFrames Joining
- DataFrames Handling missing data
Part -5 Data Visualization
Matplotlib
- Basic matplotlib plots
- Line Plots
- Bar Plots
- Pie Plots
- Scatter plots
- Histogram Plots
Seaborn
- Categorical Plots
- Bar Plots
- Box Plots
- Heatmaps Plots
- Pair Plots
- Regression Plots
Plotly – Python Plotting
- Plotly
- Geographical Plotting
- Choropleth Maps – Part 1
- Choropleth Maps – Part 2
- Choropleth Exercises
- Projects using Analysis and Visualisation
Part 6- Statistics, Probability & Business Analytics
Basic Statistics
- Data types and their measures
- Measures of Central Tendency
- Arithmetic mean
- Harmonic mean
- Geometric mean
- Median
Probability Distributions
- Intro of probability
- Conditional probability
- Normal distribution
- Uniform distribution
- Frequency distribution
- Central limit theorem
Hypothesis Testing
- Concept of Hypothesis Testing
- Statistical Methods
- Z-Test
- T-Test
- One Way Anova Test
- Two Way Anova Test
Part 7- Machine Learning
Machine Learning
- What is Machine learning?
- Overview about scikit-learn package
- Types of ML
- Basic steps of ML
- ML algorithms
- Machine learning examples
Data Preprocessing / Data Cleaning
- Dealing with missing data
- Identifying missing values
- Imputing missing values
- Drop samples with missing values
- Handling with categorical data
- Nominal and Ordinal features
KNN Classifiers
- K-Nearest Neighbours (KNN)
- KNN Theory
- KNN implementation
- KNN Project Overview and Project Solutions
Regression Based Learning
- Linear Regression Theory
- Dependent and independent Variables
- Linear Regression with Python implementation
- Multiple linear regression
- Polynomial regression
- Regularization
Logistic Regression for Classification
- Logistic Regression Theory
- Binary and multiclass classification
- Implementing titanic dataset
- Implementing iris dataset
- Sigmoid and softmax functions
- Nominal and Ordinal features
Decision Tree Classification
- Entropy and Information gain
- Intro to bagging algorithm
- Implementation with iris dataset
- Visualizing Decision Tree
- Ensemble Learning
- Random forest
Support Vector Machines
- Introduction to SVM
- Working of SVM and its uses
- Working with High Dimensional Data
- Kernel, gamma, margin
- Breast Cancer Prediction Project using SVM
Naive Bayes Algorithm
- Conditional Probability
- Overview of Naïve Bayes Algorithm
- Feature extraction
- CountVectorizer
- TfidfVectorizer
- Nominal and Ordinal features
Clustering Based Learning
- K-means Clustering Algorithm
- Elbow technique
- Silhouette coefficient
- K Means Clustering Project Overview
- K Means Clustering Project Solutions
Recommendation System
- Content based technique
- Collaborative filtering technique
- Evaluating similarity based on correlation
- Classification-based recommendations
Natural Language Processing
- Install nltk
- Tokenize words
- Tokenizing sentences
- Stop words with NLTK
- Nominal and Ordinal features
Working with OpenCV
- Basic of Computer Vision & OpenCV
- Reading and writing images
- Resizing image
- Applying image filters
- Writing text on images
Part 8- Deep Learning
Deep Learning
- What is Deep Learning?
- Deep Learning Packages
- Deep Learning Applications
- Building deep learning environment
- Installing tensor flow locally
- Understanding Google Colab
Tensor Flow Basics
- What is tensorflow?
- Tensorflow 1.x v/s tensorflow 2.x
- Variables, Constants, Placeholder
- Scalar, vector, matrix
- Operations using tensorflow
- Tensor Flow Computational graph
Artificial Neural Network
- What is Artificial Neural Network
- How neural network works?
- Perceptron
- Multilayer Perceptron
- Feedforward
- Back propagation
Activation Functions
- Linear Activation Function
- Sigmoid function
- Hyperbolic tangent function
- ReLU –rectified linear unit
Optimizers
- What does optimizers do?
- Gradient descent
- Stochastic gradient descent
- Learning rate , epoch
Building Artificial Neural Network
- Using scikit implementation
- Using tensorflow
- Understanding mnist dataset
- Initializing weights and biases
Deep Learning Optimizer
- SGD with momentum
- RMSprop
- AdaGrad
- Adam
Deep Neural Network Using Keras
- What is keras?
- Keras fundamental for deep learning
- Keras sequential model and functional api
- Saving and loading a keras model
Convolutional Neural Network
- CNN architecture
- Convolutional operations
- Pooling, stride and padding operations
- Data augmentation
Part 9- R Language
Introduction to R
- What is R
- History of R
- Features of R
- Obtaining and managing R
- Installing R
- Packages
Data Types and Objects
- Data Types
- Variables in R
- Scalars
- Vectors
- Factors
- Numbers
Data Management
- Reading Data
- Writing data
- Reading data files with tables
- Files connection
- Reading lines of Text files
- Sorting Data
Import and Export Data in R
- Importing data in to R
- CSV File
- Excel File
- Import data from text table
Control Structures and Functions
- Control statements [if,if..else,next,return]
- Loop statements [while, for, repeat]
- Functions
- Function arguments & options
Database connectivity with R
- How to install RMysql Package
- How to connect R to Mysql Database
- Operation on Mysql Queries on R
Part 10- Data Analytics using R
R LIBRARIES
- What is data analysis?
- Why R for data analysis?
- Essential R Libraries Installation and setup
- Rstudio Overview
MANAGING DATA FRAMES WITH THE DPLYR PACKAGE
- The dplyr Package
- Installing the dplyr package
- select()
- filter()
DATA CLEANING WITH THE TIDYR PACKAGE
- The tidyr Package
- Installing the tidyr package
- Installing the data.table package
- Cleaning data
Part11- Data Visualization using R
GRAPHICS AND PLOTTING USING R Basic Plotting
- Basic plots in R
- Line Plots
- Bar Plots
- Pie Plots
- Box Plots
- Scatter plots
ADVANCE PLOTTING GGPLOT 2 Visualization
- Layers of ggplot2 package
- How to install ggplot2 package
- Histogram Plots
- Scatter plots
- Bar Plots
- Box Plots
Interactive Visualizations with Plotly
- Overview of Plotly and Interactive Visualizations
- How to install Plotly Interactive Visualization Package
- Plotly Visualizations
- Cleaning data
Part 12- Core Tableau for Data Analysis
Tableau Desktop part-1
- Introduction to Tableau
- What is Tableau?
- Kinds of tableau
- Tableau architecture
- Overview to different versions
Tableau Desktop part-2
- Installation of Tableau Desktop
- Understanding tableau user interface
- Connect Tableau to Datasets
- Data analysis with tableau
- Bar Charts
Tableau Desktop part-3
- Scatter Charts
- Pie Charts
- Creating maps and setting map options
- Creating Dashboards
- Interactive Dashboards
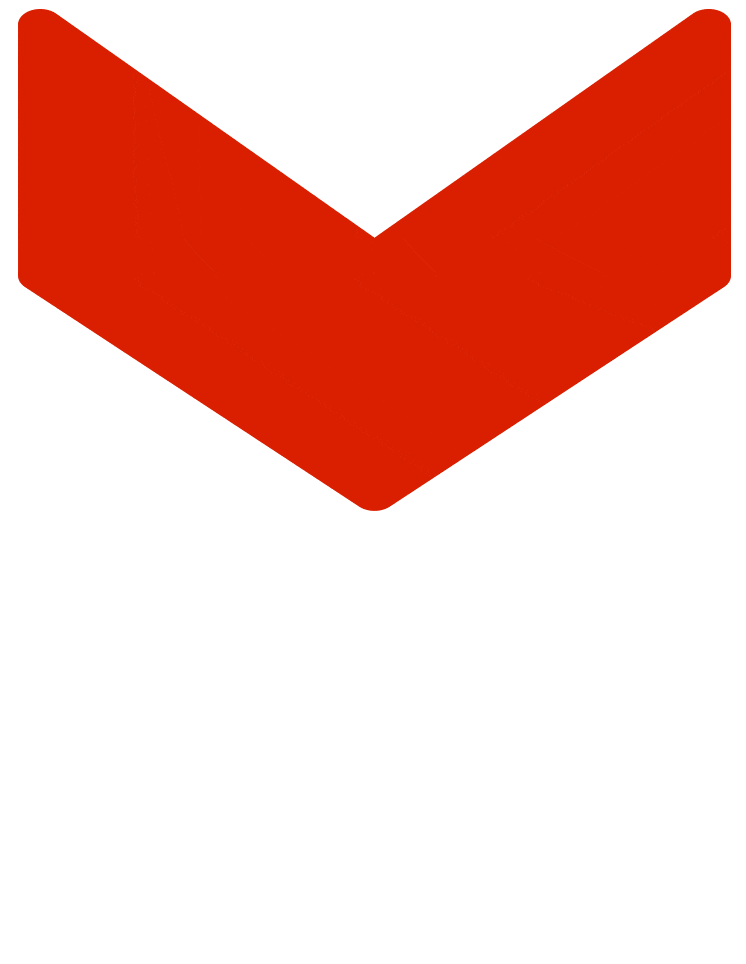